Intro
I've been developing my own little Game Engine since March 2019. Now you might think, wait 2019 and still nothing to show? Yeah well, you know how it is with side projects. You begin something and you're really motivated, and then something gets in the way. You loose focus and after months you decide to continue your project. If you look after months of doing nothing with your code you're like:
"OK - where to begin I don't recognize anything".
There are many many really good Game Engines already available. And yeah also really good Game Engines which are free to use. No payments no anything. But I wanted something different. I wanted to code it all myself. And I'm not coding this to sell the Engine or do anything particular with it. I think it's a good way to learn things. Not only to learn things, but also to use all the things you learned while studying Computer Science. And believe me, in Games you really need the stuff you learned.
And of course: I really like computer games.
But lets begin with:
A blank screen.
So you wanna draw something to the screen I guess. Yes I thought that too, I wanted to put some sick animations, characters, trees, grass on the screen let it move around, maybe throw some physics in it? And while we're on it multiplayer and 3d of course. Couldn't be that hard, could it?
Well 3d is something I still have to learn a bit and knew little about it back then. So I just decided to go with 2d. I always liked 2d games anyway, top down or sidescrollers doesn't matter. Besides who needs three dimensions when you can have two.
Back to that blank screen. How to get a blank screen to draw something on it? Luckily for us there are some really good Libraries that to the heavy lifting of creating a window for us.
There is:
- GLFW
- SDL
- GLUT (unsupported for over 20 years)
- FreeGLUT (I like how literally the first word you read is: What?) ;)
I think the majority of people either code it themselves (Big Game Engine companies) or they just use GLFW/SDL.
They are the most common. I for example used SDL. With SDL you can get your window like this: (from the SDL Wiki Page)
// Example program:
// Using SDL2 to create an application window
#include "SDL.h"
#include <stdio.h>
int main(int argc, char* argv[]) {
SDL_Window *window; // Declare a pointer
SDL_Init(SDL_INIT_VIDEO); // Initialize SDL2
// Create an application window with the following settings:
window = SDL_CreateWindow(
"An SDL2 window", // window title
SDL_WINDOWPOS_UNDEFINED, // initial x position
SDL_WINDOWPOS_UNDEFINED, // initial y position
640, // width, in pixels
480, // height, in pixels
SDL_WINDOW_OPENGL // flags - see below
);
// Check that the window was successfully created
if (window == NULL) {
// In the case that the window could not be made...
printf("Could not create window: %s\n", SDL_GetError());
return 1;
}
// The window is open: could enter program loop here (see SDL_PollEvent())
SDL_Delay(3000); // Pause execution for 3000 milliseconds, for example
// Close and destroy the window
SDL_DestroyWindow(window);
// Clean up
SDL_Quit();
return 0;
}
With GLFW like this:
#include <GLFW/glfw3.h>
int main(void)
{
GLFWwindow* window;
/* Initialize the library */
if (!glfwInit())
return -1;
/* Create a windowed mode window and its OpenGL context */
window = glfwCreateWindow(640, 480, "Hello World", NULL, NULL);
if (!window)
{
glfwTerminate();
return -1;
}
/* Make the window's context current */
glfwMakeContextCurrent(window);
/* Loop until the user closes the window */
while (!glfwWindowShouldClose(window))
{
/* Render here */
glClear(GL_COLOR_BUFFER_BIT);
/* Swap front and back buffers */
glfwSwapBuffers(window);
/* Poll for and process events */
glfwPollEvents();
}
glfwTerminate();
return 0;
}
With GLFW you have to do a little bit more OpenGL directly, where with SDL, if you're only doing 2d anyway you have nice little function calls for 2d and don't have to hassle with OpenGL, if that's not your thing. (You can use OpenGL with SDL no problems)
What those frameworks are doing for you is like opening a window, getting system events and drawing something to that window. Above in the example you see something like that:
while(true) { do_something(); }
Also called Game-Loop. Thats where the magic happens, thats where each frame gets rendered, there you do your logic of your game, almost anything.
So go on make your window. But this series is about the engine I code and what I'm doing right now.
Right now I'm in the middle of developing some GUI things.
GUI
Graphical-User-Interface. There comes a time when you need to put some text on the screen. And then you think, well now I have this Text, I need a box with text in it and ... wait how can I render text. I really need an FPS counter thats cool. And then you realize that putting text on the screen is not so trivial as it might seem. SDL can do a lot of work for you. But Buttons, TextBoxes, TextInputBoxes you have to code it all yourself. Or use a library. But we are here for the fun of learning things.
Your events also have to go only to the GUI thing you're using right now. It would be funny if you press W-A-S-D and your player moves while writing some text into an TextInput Field. I've got that all working now. It was some work, but again learned alot. But more on the GUI in the next post.
For now some screenshots of the different stages my engine went so far.
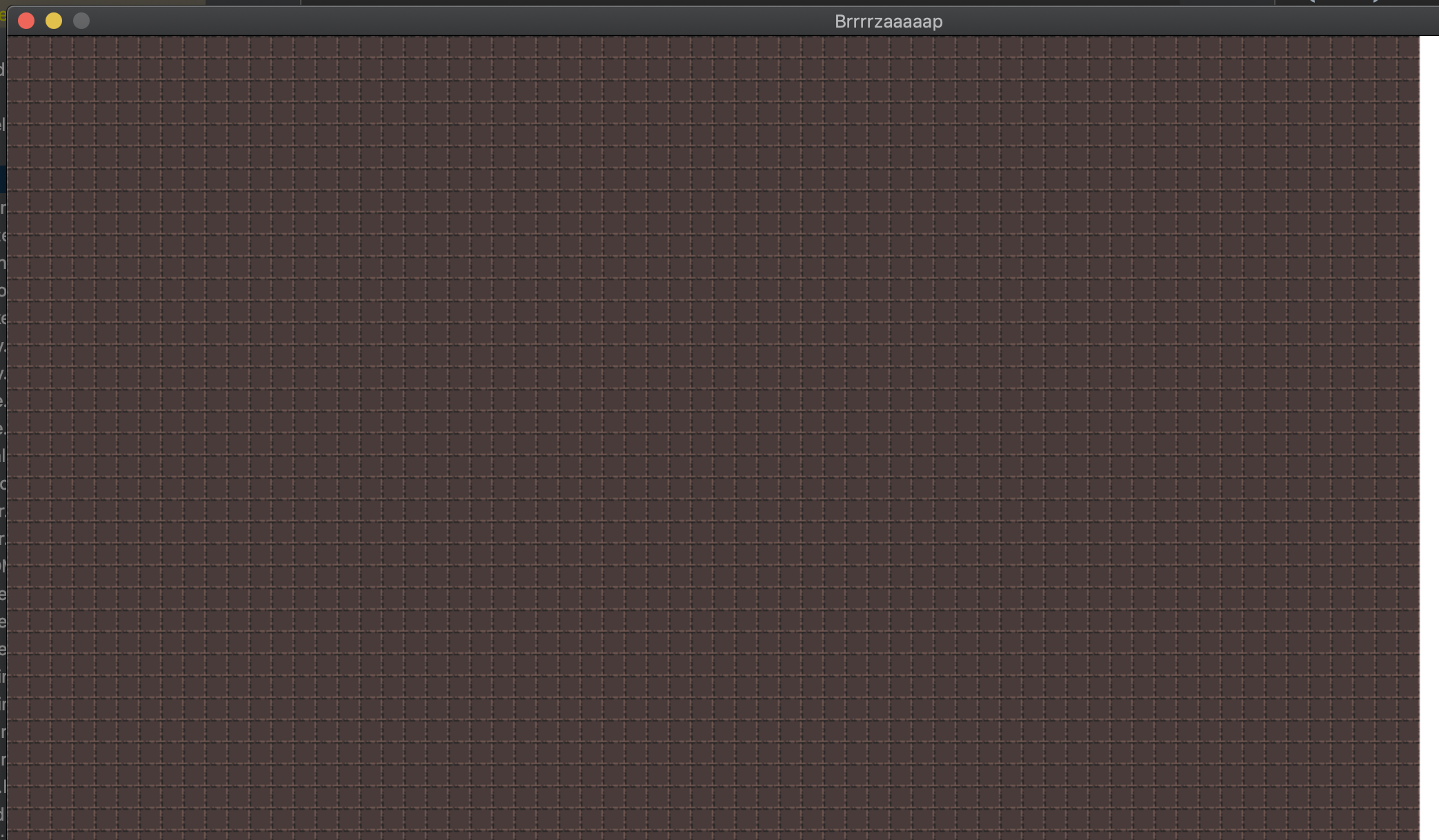
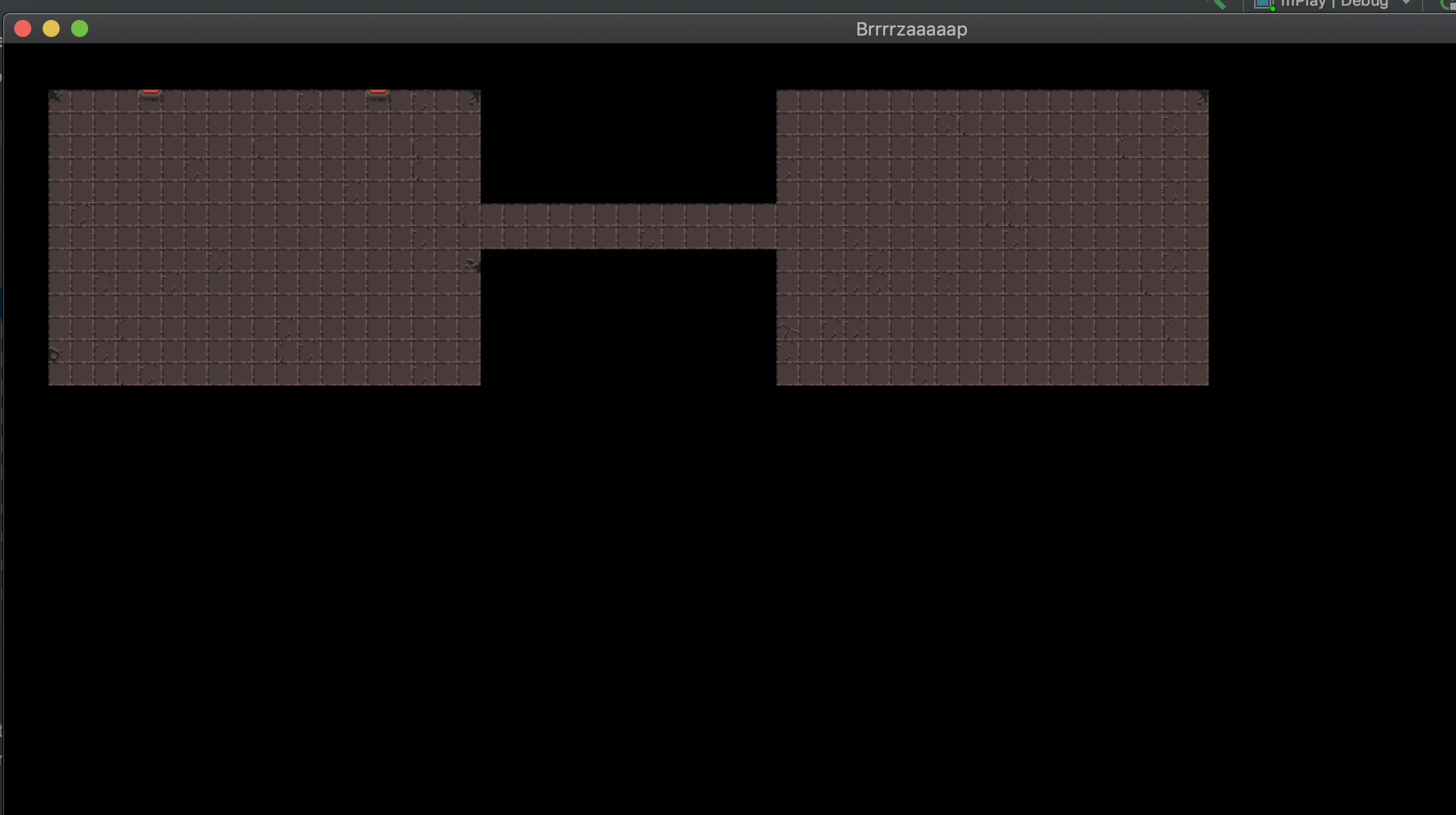
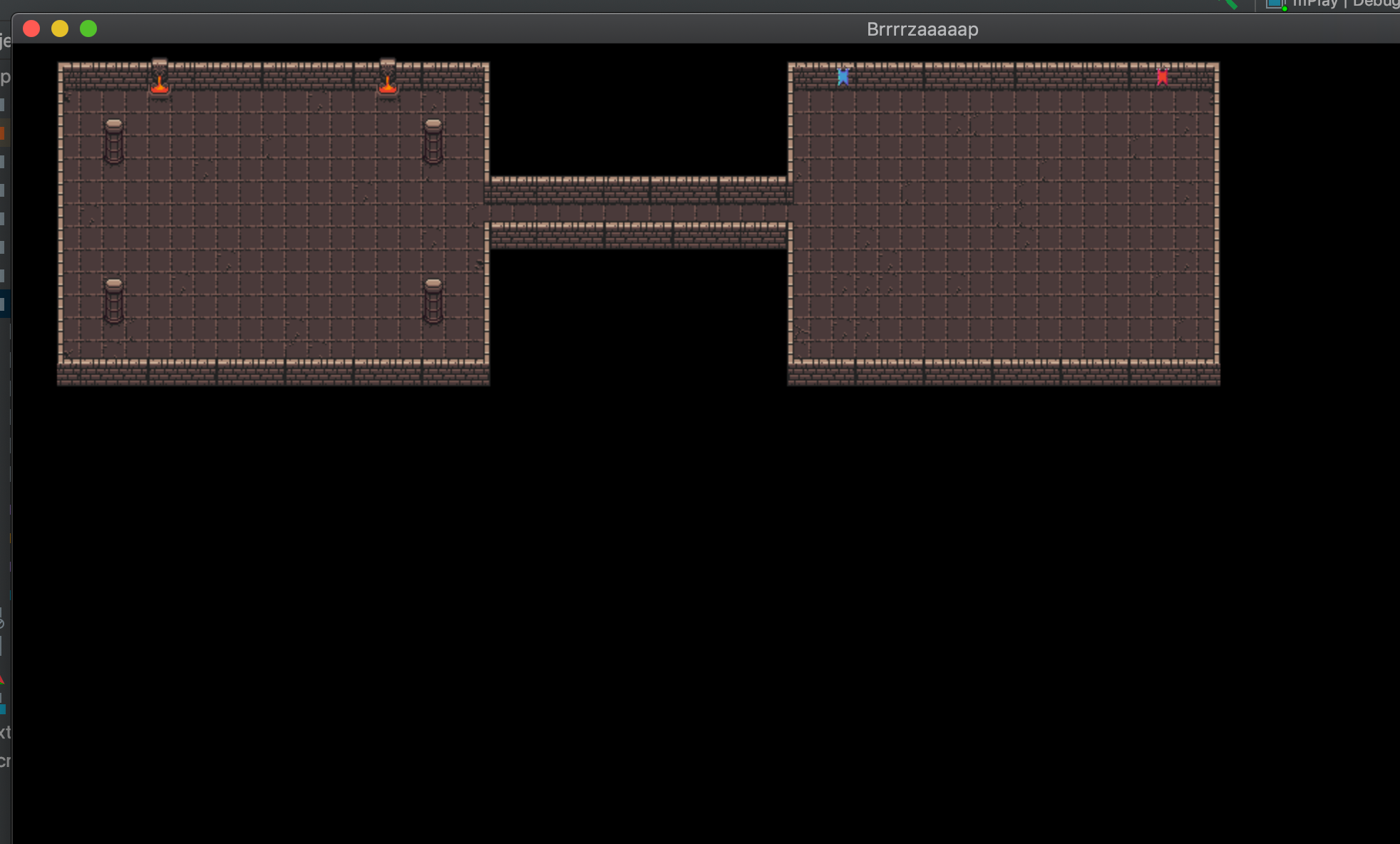
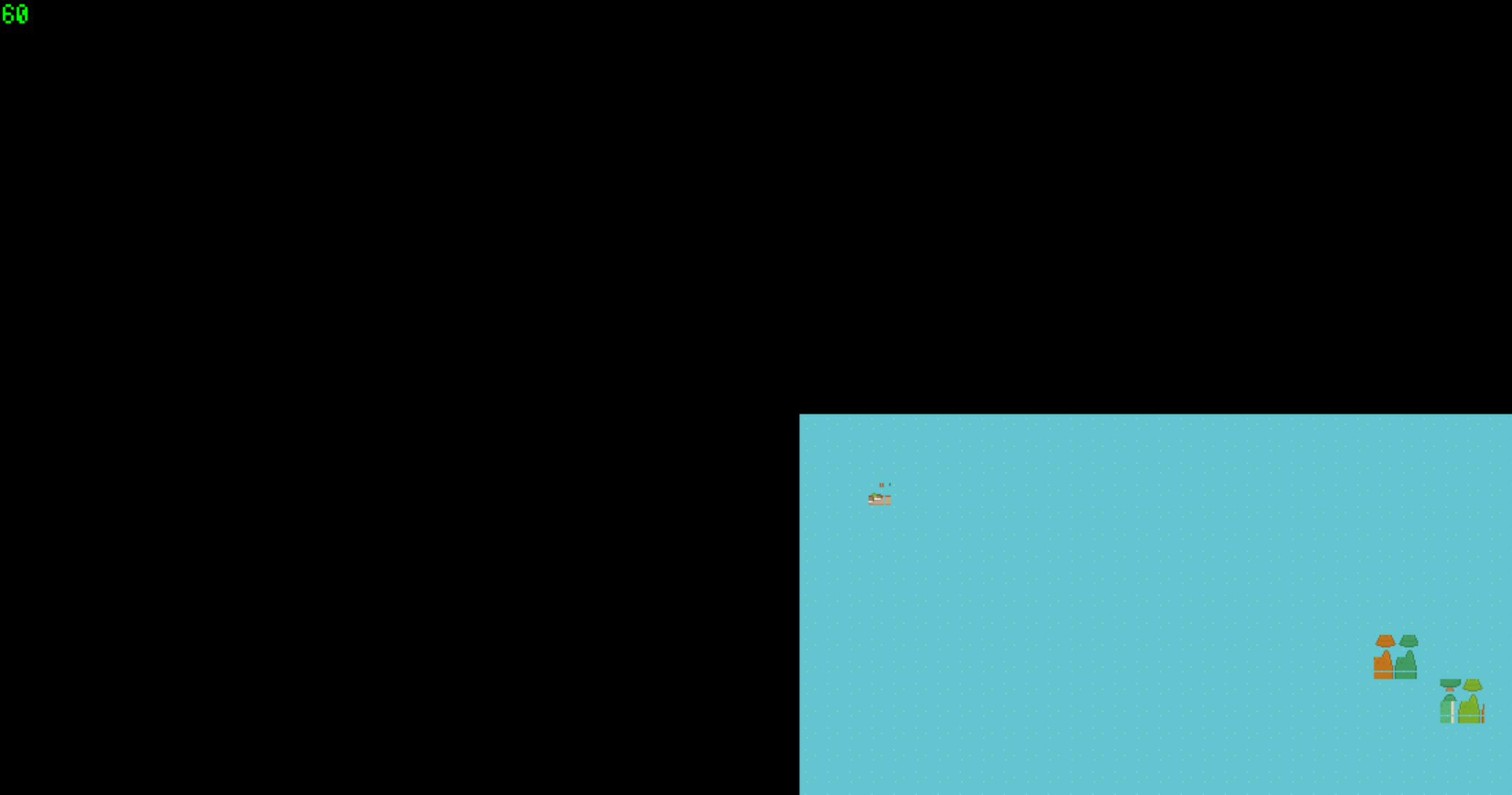
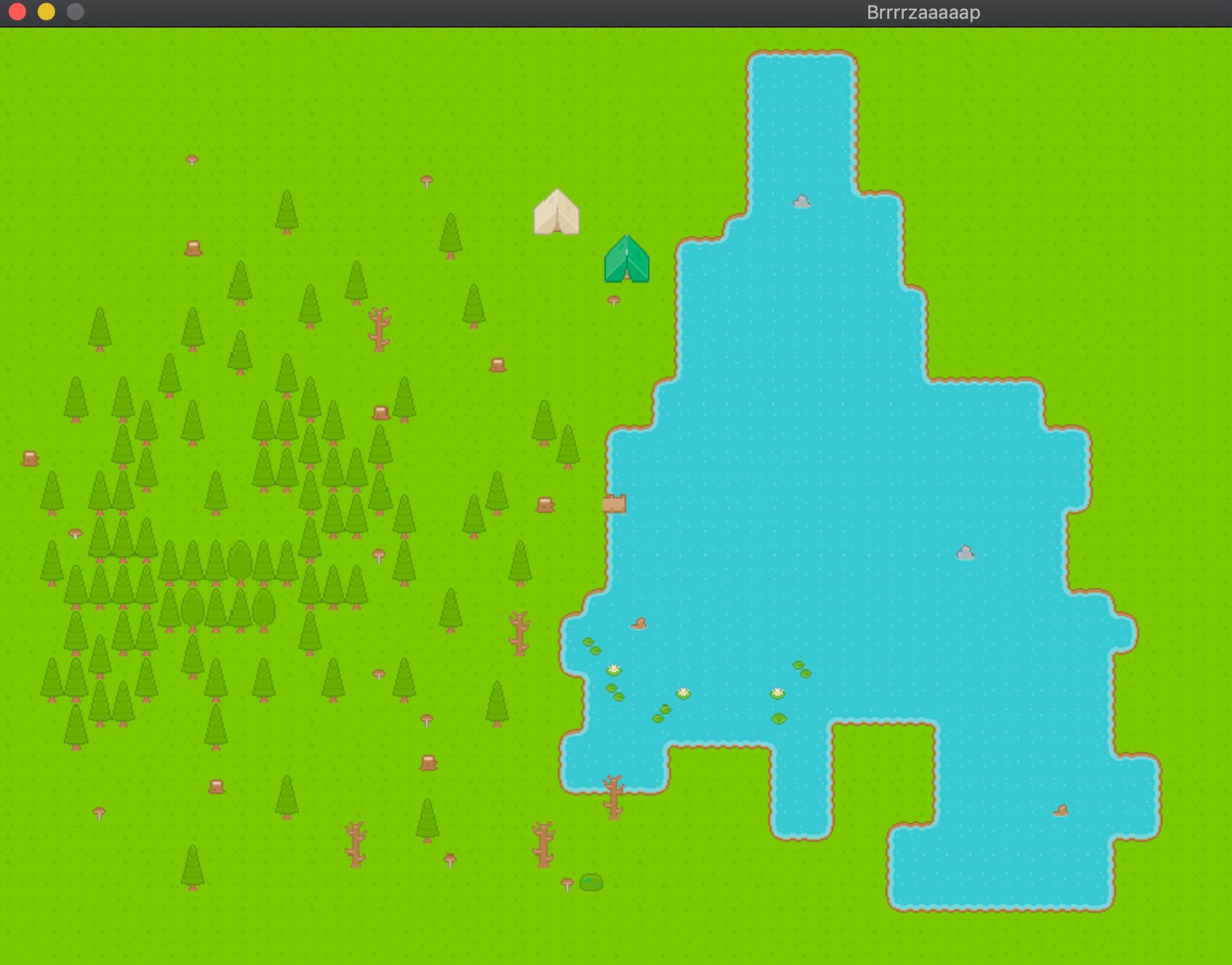
More Screenshots are coming soon. I really have to tag some git commits as "working" so my future me can easily checkout old working commits to look at some old work.
Thats it for now. Please please if you liked this, leave a comment. (If you don't liked it please go away) :D Really like to hear from some other developers, gamers, readers etc.
If you press this Button it will Load Disqus-Comments. More on Disqus Privacy: Link