The Abstract Factory belongs to the creation patterns. Objects can be created with it. It belongs to the famous twenty-threee GoF design Patterns. For example, a GUIFactory could be used to create buttons that are displayed in a window. In Windows this would possibly be done with the help of windows.h
. In Mac OS X maybe with SDL. If you are building a GUI you could use a GUIFactory like shown in the graphic below.
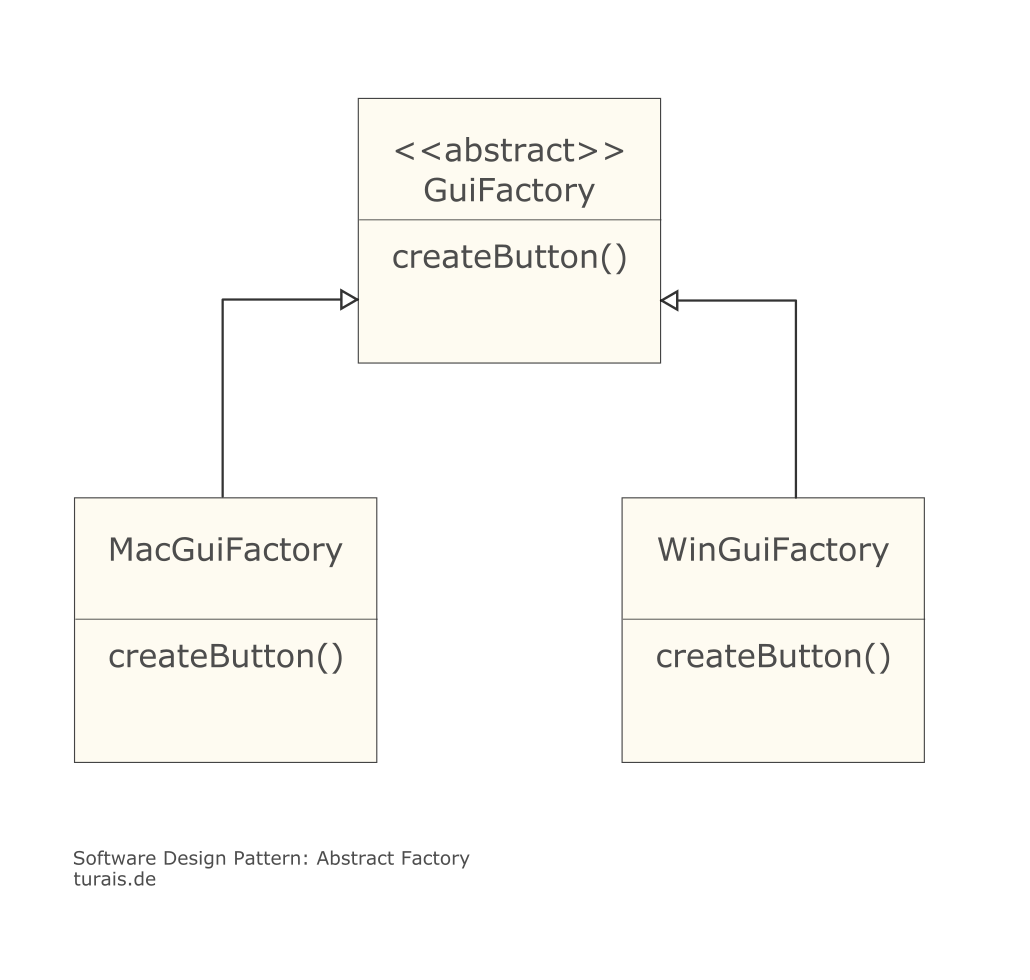
Now if you create a GuiFactory, depending on which operating system you are building your application for, you create either a MacGuiFactory or a WinGuiFactory,
like so:
GuiFactory factory;
if(MAC) {
factory = new MacGuiFactory();
} else if(WIN) {
factory = new WinGuiFactory();
}
Later on in the code you can use the factory
like this:
factory.createButton();
It should also be mentioned here that the client, i.e. the user of your library, does not import the Button class, but an abstract Button class or a higher-level class, such as GuiElement. The whole thing can be used like this:
GuiElement button = factory.createButton();
Another advantage is that the whole factory can be exchanged to serve your purpose. In Windows you're using the WinGuiFactory and on MacOSX for example another Factory. The GUI Code itself is abstracted enough to focus on the important things, like how a Button is created with which elements and so on. In your specific factory you define things like how exactly on that operating system the button is created.
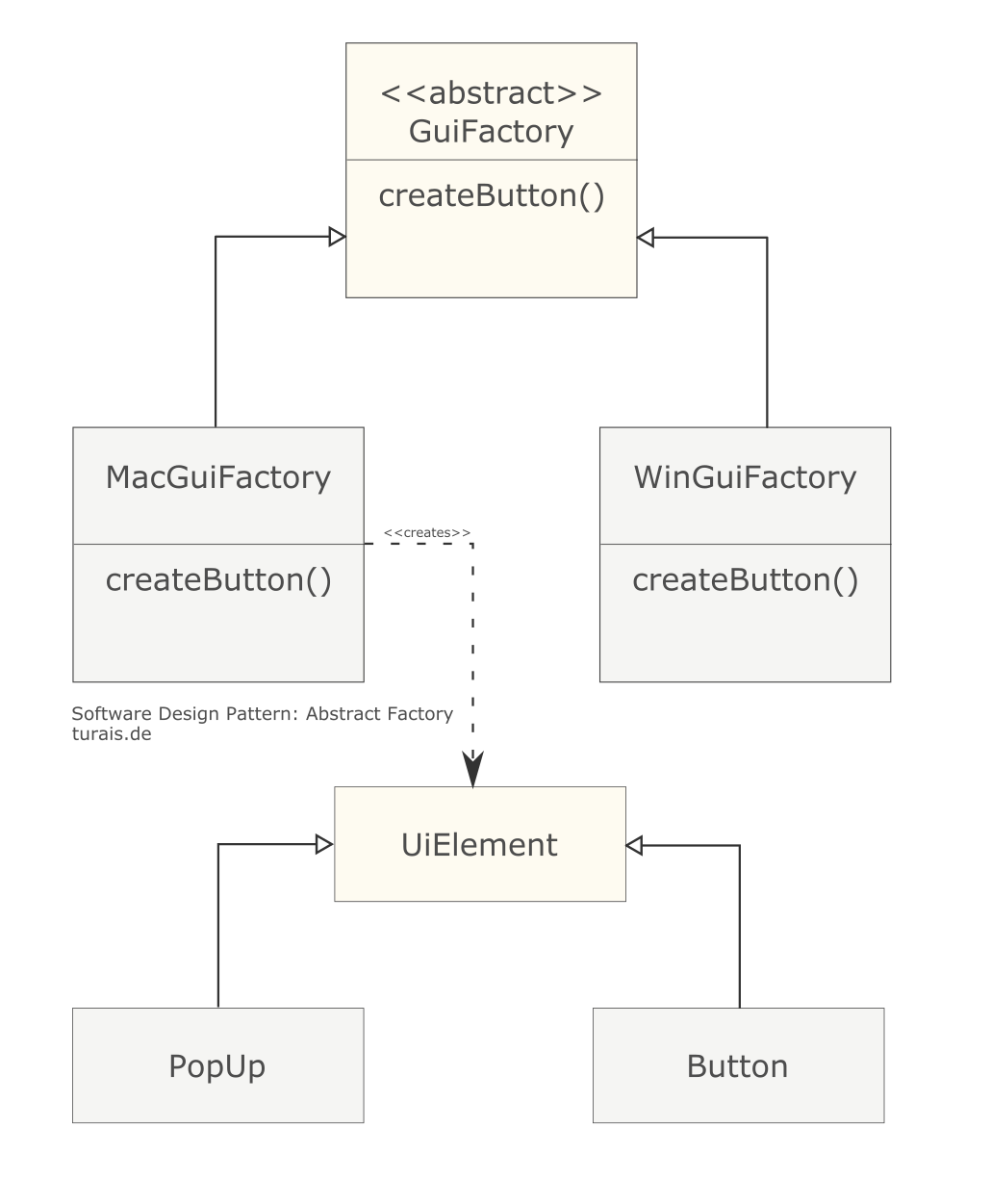
In the above UML example the MacGuiFactory creates an UIElement in this case this would be an specific UiElement for Mac OS X. A more advanced example can be seen here: https://en.wikipedia.org/wiki/Abstract_factory_pattern#/media/File:Abstract_factory.svg
A user would do something like this:
UiElement popup = factory.createPopup();
UiElement abort_button = factory.createButton();
UiElement ok_button = factory.createButton();
Book recommendation
Design Patterns - Elements of Reusable Object-Orientated Software
If you press this Button it will Load Disqus-Comments. More on Disqus Privacy: Link